Over the years, we’ve explored different state management solutions in Flutter, including Provider, Riverpod and Bloc. While each brings its own strengths to the table, they also come with limitations. Today we’re taking a closer look at the two most popular options – Provider ad Riverpod – examining their strengths, weaknesses, and how to decide which one to use.
Provider:
It is built on top of the InheritedWidget. Provider is a popular state management solution in Flutter. It is straightforward yet effective. It uses dependency injection, which lets you effectively feed values to different widgets in your application. It uses the Inherited Widget’s capacity to disperse data automatically across the widget tree, guaranteeing that relevant widgets are updated each time the state is modified.
Key features of Provider:
- React Ability: Widgets automatically rebuild when the state changes.
- Simple State Management: Provides a simple way to manage and share state between widgets.
- Dependency Injection: Facilitates the injection of dependencies into widgets.
Limitations:
- Dependency on the Context: Requires a BuildContext to access the providers which can complicate writing tests and code structure.
- Complexity with Dependencies: Managing dependencies between providers can become tough in large applications.
Example of how Provider works:
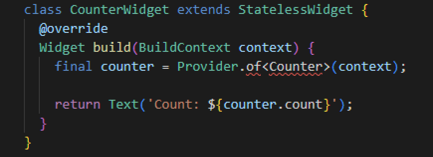
In the code above, we define a Counter class that extends ChangeNotifier, offerring state management capabilities. We then wrap the CounterWidget with ChangeNotifierProvider and utilize Provider. of <Counter>(context) within CounterWidget to access the counter instance.
RiverPod
RiverPod, on the other hand, is a newer state management solution. It addresses some of the limitations of Provider and introduces a more declarative and testable approach to state management in Flutter. RiverPod focuses on the concept of providers as first-class citizens, making is easier to read, write, and organise your code.
Key features of RiverPod:
- Enhanced performance by providing more control over state updates, reducing unnecessary rebuilds.
- Built in asynchronous support through ‘AsyncValue’, handling loading, error and completed states.
- Better testing capabilities as it does not rely on the BuildContext, making writing tests simpler.
Limitations:
- Limited Documentation for Advanced Use Cases: Although the basic documentation for RiverPod is quite good, some advanced use cases and best practices might not be as well-documented.
- Verbose Syntax can lead to more boilerplate code, especially for smaller projects.
Example of how RiverPod works:
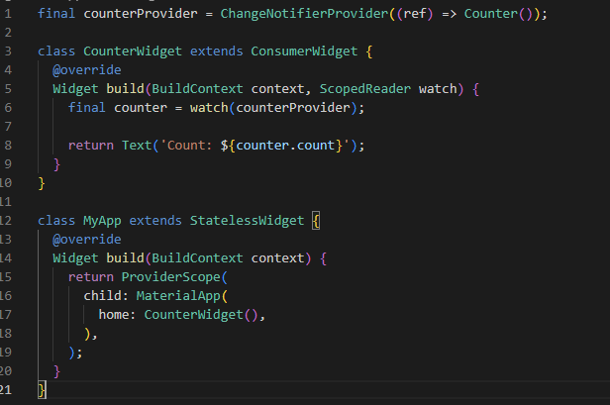
In the above code, we define a counter Provider using the ChangeNotifierProvider constructor and provide the Counter instance. Inside the CounterWidget, we use watch(counterProvider) to access the counter instance. RiverPod’s approach simplifies the code by removing the need for BuildContext and automatically handling the provider dependencies.
Final Thoughts:
While both state management solutions are great choices, there are clear strengths and weaknesses which make them each have a very specific use case.
If you are building a smaller project with simpler state management requirements, Provider is the perfect choice. Its setup is easy and is well-suited for smaller applications with a limited number of providers and widgets.
On the other hand, RiverPod is more suited for larger-scale applications that requires better organisation and testing capabilities.